With YouTube there is no easy url you can use like http://youtube.com/images/[username] which can return the users current image. The only way to get the users image is to call one of YouTube api feeds https://gdata.youtube.com/feeds/api/users/[username]
The image url returned is only of the current image, so if the user changes there profile image, then you will keep displaying the old image, so you can’t even just store this value as you need to check the feed each time. But to call this url and process it server side will consumer server resource and delay the time that the page can be sent to the user. Best to make the loading of the picture happen client side via a little bit of JavaScript.
The YouTube api call http://gdata.youtube.com/feeds/api/users/[username] can be customised to return only the fields you need. e.g.
http://gdata.youtube.com/feeds/api/users/[username]?fields=yt:username,media:thumbnail,title
It can then be further customised to return json and have it wrapped inside a script tag.
e.g. http://gdata.youtube.com/feeds/api/users/[username]?fields=yt:username,media:thumbnail,title&alt=json-in-script&format=5
Next you can add in a callback script that should be called. e.g.
http://gdata.youtube.com/feeds/api/users/[username]?fields=yt:username,media:thumbnail,title&alt=json-in-script&format=5&callback=showYouTubeProfileInfo
This will return a .js file which will contain the data values you needed with a function call to the name you specified in the callback field, making it easy to process the returned information. Example:
// API callback
showYouTubeProfileInfo({"version":"1.0","encoding":"UTF-8","entry":{"xmlns":"http://www.w3.org/2005/Atom","xmlns$media":"http://search.yahoo.com/mrss/","xmlns$yt":"http://gdata.youtube.com/schemas/2007","title":{"$t":"Daniel Mitchell","type":"text"},"media$thumbnail":{"url":"http://lh5.googleusercontent.com/-zjqJ1i7r8WU/AAAAAAAAAAI/AAAAAAAAAAA/yeOkGGM63SM/s88-c-k/photo.jpg"},"yt$username":{"$t":"[username]"}}});
Putting that all together, place the following code into the head of the document.
<script type="text/javascript">
function showYouTubeProfileInfo(data)
{
var name= data.entry.yt$username.$t;
var url = data.entry.media$thumbnail.url;
var title = data.entry.title.$t;
document.getElementById('userprofile').innerHTML = "<img src='"+ url +"'/> " + title;
}
</script>
Then in the body place the following code:
<div id="userprofile"></div>
<script type="text/javascript" src="https://gdata.youtube.com/feeds/api/users/[username]?fields=yt:username,media:thumbnail,title&alt=json-in-script&format=5&callback=showYouTubeProfileInfo">
</script>
That’s it, just change [username] to the username you want to get the details for and you will have a client side display of the YouTube users info.
If it all works it should display something a little like this:
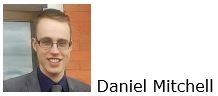